The basics of ASP.NET Core [EP2/2]
ASP.NET Core มีปัจจัยพื้นฐานที่สำคัญที่ควรมุ่งเน้นเพื่อให้เราเข้าใจและนำไปใช้ในการพัฒนาโปรแกรมอย่างมีประสิทธิภาพ
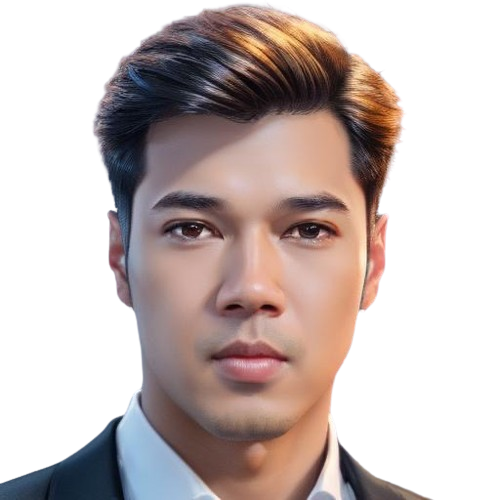
The basics of ASP.NET Core [EP2/2]
ASP.NET Core มีปัจจัยพื้นฐานที่สำคัญที่ควรมุ่งเน้นเพื่อให้เราเข้าใจและนำไปใช้ในการพัฒนาโปรแกรมอย่างมีประสิทธิภาพ ดังนี้
ยังไม่ได้อ่าน EP1 กดตรงนี้ The basics of ASP.NET Core [EP1/2]
6. การทำงานกับ API
การสร้างและใช้งาน Web API ใน ASP.NET Core มีขั้นตอนที่ต้องปฏิบัติตามเพื่อสร้าง API ที่มีประสิทธิภาพ และมีการจัดการ Request, Response และการจัดการข้อผิดพลาด Error Handling ได้อย่างเหมาะสม นี่คือขั้นตอนหลักที่ควรทราบ
6.1 สร้าง Web API Controller: สร้าง Controller โดยให้สืบทอดจาก ControllerBase และใช้ [ApiController] attribute
[ApiController]
[Route("api/[controller]")]
public class ProductsController : ControllerBase
{
// actions will be defined here
}
6.2 การกำหนด Route และ HTTP Methods: ใช้ attribute [Route] ในการกำหนดเส้นทางของ API และใช้ attribute [HttpGet], [HttpPost], [HttpPut], [HttpDelete] เพื่อกำหนด HTTP methods ที่ต้องการ
[HttpGet]
public ActionResult<IEnumerable<Product>> GetProducts()
{
// implementation for GET
}
[HttpPost]
public ActionResult<Product> CreateProduct(Product newProduct)
{
// implementation for POST
}
6.3 การจัดการ Request: ใน Actions สามารถใช้ parameters เพื่อรับข้อมูลจาก Request Body, Query String, Path หรือ Header ได้
[HttpPost]
public ActionResult<Product> CreateProduct([FromBody] Product newProduct)
{
// implementation for POST with request body
}
6.4 การจัดการ Response: ใช้ ActionResult ในการสร้าง Response ที่สามารถตอบกลับได้หลายแบบ เช่น Ok, CreatedAtAction, NotFound, BadRequest และอื่น ๆ
[HttpGet]
public ActionResult<IEnumerable<Product>> GetProducts()
{
var products = _repository.GetProducts();
return Ok(products);
}
6.5 การจัดการข้อผิดพลาด Error Handling: สามารถใช้ attribute [ProducesResponseType] เพื่อกำหนด Response Type สำหรับ error และใช้ middleware สำหรับจัดการ error ในระดับแอปพลิเคชัน
[HttpGet]
[ProducesResponseType(StatusCodes.Status200OK)]
[ProducesResponseType(StatusCodes.Status404NotFound)]
public ActionResult<Product> GetProduct(int id)
{
var product = _repository.GetProductById(id);
if (product == null)
{
return NotFound();
}
return Ok(product);
}
6.6 การใช้ Dependency Injection: เพื่อเข้าถึง services หรือ repositories ให้ Inject ใน constructor ของ Controller
private readonly IProductRepository _repository;
public ProductsController(IProductRepository repository)
{
_repository = repository;
}
6.7 การทดสอบ API: ใช้ tools หรือ frameworks ที่รองรับการทดสอบ API เพื่อตรวจสอบความถูกต้องและประสิทธิภาพของ API ที่สร้าง
การสร้างและใช้งาน Web API ใน ASP.NET Core นอกจากนี้ยังสามารถใช้ Middleware เพื่อกำหนดกระบวนการตรวจสอบและจัดการ Request และ Response ต่าง ๆ ได้ตามความต้องการของแอปพลิเคชัน
7. การทำงานกับ API
Authentication และ Authorization เป็นเรื่องสำคัญที่ช่วยให้ปกป้องแอปพลิเคชันของเราจากการเข้าถึงที่ไม่ได้รับอนุญาต ใน ASP.NET Core การจัดการ Authentication และ Authorization นั้นมีความยืดหยุ่นและสามารถทำได้หลายวิธีตามความต้องการของแอปพลิเคชัน นี่คือวิธีการทำ Authentication และ Authorization ใน ASP.NET Core
7.1 Authentication
7.1.1 การกำหนด Identity Middleware: Identity Middleware ใน ASP.NET Core จะช่วยจัดการกระบวนการ Authentication ให้เพิ่ม middleware นี้ใน Configure ของ Startup
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
// ...
app.UseAuthentication();
// ...
}
7.1.2 การกำหนด Authentication Scheme: ใน ConfigureServices กำหนด Authentication Scheme ที่ต้องการใช้งาน
public void ConfigureServices(IServiceCollection services)
{
// ...
services.AddAuthentication("MyAuthenticationScheme")
.AddCookie("MyAuthenticationScheme", options =>
{
// configure cookie options
});
// ...
}
7.1.3 การให้บริการใน Controllers: Inject SignInManager และ UserManager เพื่อให้ Controllers สามารถทำงานกับ Identity ได้
public class AccountController : Controller
{
private readonly SignInManager<ApplicationUser> _signInManager;
private readonly UserManager<ApplicationUser> _userManager;
public AccountController(
SignInManager<ApplicationUser> signInManager,
UserManager<ApplicationUser> userManager)
{
_signInManager = signInManager;
_userManager = userManager;
}
// ...
}
7.1.4 Login and Logout Actions: สร้าง Actions สำหรับ Login และ Logout ใน Controller
[HttpPost]
public async Task<IActionResult> Login(LoginViewModel model)
{
// validate and authenticate user
var result = await _signInManager.PasswordSignInAsync(model.Email, model.Password, model.RememberMe, lockoutOnFailure: false);
if (result.Succeeded)
{
return RedirectToAction("Index", "Home");
}
// authentication failed
ModelState.AddModelError(string.Empty, "Invalid login attempt");
return View(model);
}
[HttpPost]
public async Task<IActionResult> Logout()
{
await _signInManager.SignOutAsync();
return RedirectToAction("Index", "Home");
}
7.2 Authorization
7.2.1 การกำหนด Policy: ใน ConfigureServices กำหนด Policy สำหรับ Authorization
public void ConfigureServices(IServiceCollection services)
{
// ...
services.AddAuthorization(options =>
{
options.AddPolicy("RequireAdminRole", policy => policy.RequireRole("Admin"));
});
// ...
}
7.2.2 ใช้ Attribute ใน Controllers: ใช้ [Authorize] attribute หรือกำหนด Policy ใน Action เพื่อปกป้องการเข้าถึง
[Authorize(Policy = "RequireAdminRole")]
public class AdminController : Controller
{
// actions that require admin role
}
7.2.3 ตรวจสอบ Roles ใน Controllers: ใน Controllers สามารถใช้ User property ใน HttpContext ในการตรวจสอบ Roles
public class SomeController : Controller
{
public IActionResult SomeAction()
{
if (User.IsInRole("Admin"))
{
// perform action for Admin
}
.else
{
// unauthorized
return Forbid();
}
}
}
การทำ Authentication และ Authorization ใน ASP.NET Core ช่วยให้เราปกป้องแอปพลิเคชันของเราไม่ให้ผู้ไม่ได้รับอนุญาตเข้าถึงทรัพยากรที่สำคัญ และช่วยในการรักษาความปลอดภัยของข้อมูลและการทำงานของแอปพลิเคชัน
8. การทำ Unit Testing
การเขียน Unit Test เป็นส่วนสำคัญในการพัฒนาซอฟต์แวร์เพื่อให้แน่ใจว่าโค้ดของเรามีประสิทธิภาพ ทำงานได้ตามที่คาดหวัง และปราศจากข้อผิดพลาด นี้คือขั้นตอนที่สามารถทำเพื่อเขียน Unit Test สำหรับ ASP.NET Core Application
8.1 เลือก Framework ทดสอบ: ใน ASP.NET Core มีหลาย Framework ทดสอบที่สามารถใช้งานได้ เช่น xUnit, MSTest, NUnit เลือก Framework ที่เราสะดวกในการใช้และสอดคล้องกับทีมของเรา
8.2 สร้าง Project ทดสอบ: สร้างโปรเจคทดสอบ Test Project ในโปรเจคของเรา เป็นไฟล์ .csproj ที่มีลักษณะเฉพาะของ Test Project
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netcoreapp3.1</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.NET.Test.Sdk" Version="16.10.0" />
<PackageReference Include="xunit" Version="2.4.1" />
<PackageReference Include="xunit.runner.visualstudio" Version="2.4.1" />
</ItemGroup>
</Project>
8.3 เขียน Unit Test: เขียน Unit Test โดยใช้ Assert ในการตรวจสอบผลลัพธ์ของโค้ด
public class Calculator
{
public int Add(int a, int b)
{
return a + b;
}
}
public class CalculatorTests
{
[Fact]
public void Add_ReturnsCorrectSum()
{
// arrange
var calculator = new Calculator();
// act
var result = calculator.Add(2, 3);
// assert
Assert.Equal(5, result);
}
}
8.4 ใช้ Dependency Injection ใน Unit Test: ใน ASP.NET Core สามารถใช้ Dependency Injection ใน Unit Test ได้โดยใช้ ServiceProvider
public class MyServiceTests
{
[Fact]
public void MyMethod_ReturnsExpectedResult()
{
// arrange
var serviceProvider = new ServiceCollection()
.AddSingleton<IMyDependency, MyDependencyStub>()
.BuildServiceProvider();
var myService = new MyService(serviceProvider);
// act
var result = myService.MyMethod();
// assert
Assert.Equal("ExpectedResult", result);
}
}
8.5 ใช้ Mocking Framework: สำหรับการทดสอบที่มีการเรียกใช้ external dependencies ควรใช้ Mocking Framework เช่น Moq เพื่อจำลองการทำงานของ dependencies
public class MyServiceTests
{
[Fact]
public void MyMethod_CallsDependencyMethod()
{
// arrange
var mockDependency = new Mock<IMyDependency>();
mockDependency.Setup(d => d.MethodToBeCalled()).Verifiable();
var myService = new MyService(mockDependency.Object);
// act
myService.MyMethod();
// assert
mockDependency.Verify(d => d.MethodToBeCalled(), Times.Once);
}
}
8.6 ใช้ Test Runner: เมื่อเขียน Unit Test เสร็จ ให้ใช้ Test Runner ที่ได้เลือก (Visual Studio Test Explorer, dotnet test, Rider, etc.) เพื่อรัน Test และตรวจสอบผลลัพธ์
การเขียน Unit Test เป็นวิธีที่ดีในการทดสอบโค้ดของเราในทุกระดับ ช่วยในการค้นหาและแก้ไขข้อผิดพลาด รักษาความน่าเชื่อถือในโค้ด และช่วยในการพัฒนาแอปพลิเคชันให้มีประสิทธิภาพ
9. ความปลอดภัย
ความปลอดภัยเป็นปัจจัยสำคัญที่ไม่ควรละเลยในการพัฒนาแอปพลิเคชัน การป้องกันต่อการโจมตีที่เป็นที่รู้จักเป็นสิ่งสำคัญเพื่อให้แน่ใจว่าแอปพลิเคชันของเรามีระบบความปลอดภัยที่เพียงพอ นี่คือบางเรื่องที่ควรให้ความสำคัญเมื่อพัฒนาแอปพลิเคชัน
-
9.1 การป้องกันการโจมตี CSRF (Cross-Site Request Forgery)
- การใช้ CSRF Token: ในแต่ละรีเควสที่มีผลกระทบที่สำคัญ เราควรให้ CSRFToken เพื่อป้องกันการโจมตี โดย CSRF Token นี้จะถูกสร้างขึ้นและแนบไปกับแต่ละรีเควส และเซิร์ฟเวอร์จะตรวจสอบความถูกต้องของ Token ก่อนที่จะดำเนินการใดๆ ต่อไป
- SameSite Cookies Attribute: การใช้ SameSite attribute ใน Cookies ช่วยลดความเสี่ยงของการโจมตี CSRF โดยการกำหนดค่าเป็น "Strict" หรือ "Lax" ซึ่งจะจำกัดการส่ง Cookie จาก cross-site requests
-
9.2 การป้องกันการโจมตี XSS (Cross-Site Scripting)
- Input Validation: ทำการตรวจสอบและกันการป้องกันข้อมูลที่รับเข้ามา เพื่อป้องกัน XSS ผ่านทาง input fields หรือ URL parameters
- Output Encoding: สำหรับข้อมูลที่แสดงผล (output) ให้ใช้ output encoding เพื่อป้องกันการแทรกโค้ดที่ไม่ปลอดภัย
- Content Security Policy (CSP): การใช้ CSP header ที่กำหนดนโยบายการโหลดแหล่งทรัพยากรในเว็บไซต์ช่วยลดความเสี่ยงของการโจมตี XSS
-
9.3 การจัดการรหัสผ่าน
- Password Hashing: ในการเก็บรหัสผ่าน ควรใช้การแฮช (hashing) โดยใช้ฟังก์ชันแฮช เช่น bcrypt, Argon2 ไม่ควรใช้การเก็บรหัสผ่านแบบเป็นข้อความ (plaintext)
- การใช้ Salt: การใช้ salt ร่วมกับการแฮชเพื่อป้องกันการโจมตีด้วยตารางรหัสผ่าน (rainbow table)
- Password Complexity Policy: ให้กำหนดนโยบายความซับซ้อนในการตั้งรหัสผ่าน เพื่อให้ผู้ใช้สร้างรหัสผ่าน
-
9.4 การใช้ HTTPS
- การใช้ SSL/TLS: การให้บริการผ่าน HTTPS ช่วยป้องกันการดักจับข้อมูลที่ถูกส่งระหว่างเครื่องให้กับเครื่อง
- Secure Cookies: การใช้ secure attribute ใน Cookies เพื่อให้มีการส่ง Cookies ไปที่เซิร์ฟเวอร์ผ่านช่องทางที่ปลอดภัย (HTTPS) เท่านั้น
- HTTP Strict Transport Security (HSTS): การใช้ HSTS header เพื่อกำหนดให้เบราว์เซอร์ทำงานผ่านทาง HTTPS เท่านั้น ลดความเสี่ยงจากการโจมตี
การป้องกันทั้ง CSRF, XSS การจัดการรหัสผ่าน และการใช้ HTTPS เป็นเรื่องที่จำเป็นเพื่อให้แน่ใจว่าแอปพลิเคชันของเรามีระบบความปลอดภัยที่มีประสิทธิภาพ
10. การทำงานกับ Frontend
การใช้ ASP.NET Core ในบทบาทของ Backend สำหรับ Frontend ที่สร้างด้วย JavaScript Frameworks เช่น React, Angular, หรือ Vue มีแนวคิดที่คล้ายกันทั้งหมดเนื่องจากการสร้างแอปพลิเคชันที่แยกออกเป็น Frontend และ Backend เป็นแนวทางที่ทันสมัยและมีประสิทธิภาพ นี้คือขั้นตอนที่สำคัญและแนวคิดที่ควรรู้เมื่อใช้ ASP.NET Core ในบทบาท Backend
10.1 สร้าง Web API ด้วย ASP.NET Core
Controller สำหรับ API: สร้าง Controllers ที่ให้บริการผ่านทาง API โดยใช้ ControllerBase และประกาศ Actions ที่จะทำงานเมื่อรับ Request
[ApiController]
[Route("api/[controller]")]
public class ProductsController : ControllerBase
{
[HttpGet]
public ActionResult<IEnumerable<Product>> GetProducts()
{
// implementation for GET
}
[HttpPost]
public ActionResult<Product> CreateProduct(Product newProduct)
{
// implementation for POST
}
}
การใช้ Dependency Injection: ใช้ Dependency Injection เพื่อเข้าถึง services ที่จำเป็น เช่น DbContext สำหรับ Entity Framework, repositories หรือ services อื่น ๆ
public class ProductsController : ControllerBase
{
private readonly IProductService _productService;
public ProductsController(IProductService productService)
{
_productService = productService;
}
// actions will use _productService
}
10.2 การสร้างและจัดการ Middleware
CORS Middleware: กำหนด CORS policy เพื่ออนุญาตหรือปฏิเสธการเข้าถึง API จาก domain อื่น
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(options =>
{
options.AddPolicy("AllowAll", builder =>
{
builder.AllowAnyOrigin().AllowAnyMethod().AllowAnyHeader();
});
});
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseCors("AllowAll");
}
10.3 สร้างและให้บริการ API Documentation
Swagger/OpenAPI: ใช้ Swagger หรือ OpenAPI เพื่อสร้างเอกสาร API ที่สามารถใช้เพื่อทดสอบและทำความเข้าใจ API
public void ConfigureServices(IServiceCollection services)
{
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "My API", Version = "v1" });
});
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseSwagger();
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1");
});
}
10.4 การสร้างและให้บริการ Authentication
JWT Authentication: การใช้ JSON Web Token (JWT) เพื่อรับรองตัวตนและสร้าง token สำหรับการทำงานต่อไป
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = Configuration["Jwt:Issuer"],
ValidAudience = Configuration["Jwt:Audience"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:SecretKey"]))
};
});
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseAuthentication();
}
10.5 การจัดการและควบคุมการเข้าถึง
Role-Based Authorization: กำหนดบทบาทและให้บริการ Authorization โดยใช้ Role-based หรือ Policy-based Authorization
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthorization(options =>
{
options.AddPolicy("AdminOnly", policy => policy.RequireRole("Admin"));
});
}
[Authorize(Policy = "AdminOnly")]
public class AdminController : ControllerBase
{
// actions for admin role
}
10.6 การจัดการ Error
Global Error Handling: การใช้ Middleware เพื่อจัดการ error ระดับ application และส่ง response ที่เหมาะสม
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
.else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
}
10.7 การสร้างและให้บริการ Logging
Logging Middleware: การใช้ Middleware หรือ Logging Framework เพื่อบันทึกข้อมูลการทำงานของระบบ
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILogger<Startup> logger)
{
app.Use(async (context, next) =>
{
logger.LogInformation($"Request: {context.Request.Path}");
await next.Invoke();
});
}
10.8 การสร้างและให้บริการ Background Services
Background Services: การใช้ Background Services เพื่อประมวลผลงานที่ต้องทำต่อเนื่องหลังจากการร้องขอหรือทำงานอื่น ๆ
public class MyBackgroundService : BackgroundService
{
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
.while (!stoppingToken.IsCancellationRequested)
{
// do background work
await Task.Delay(TimeSpan.FromMinutes(15), stoppingToken);
}
}
}
-
10.9 การทำ Load Balancing และ Scaling
- Load Balancing: การใช้ Load Balancer เพื่อแบ่งการจัดการ traffic ระหว่างหลาย instances ของ Backend เพื่อเพิ่มประสิทธิภาพและความเสถียร
-
10.10 การทำ Unit Testing และ Integration Testing
- การทดสอบ: การใช้ Frameworks ทดสอบ เช่น xUnit, MSTest เพื่อทดสอบฟังก์ชันและระบบ
-
10.11 การเพิ่มความปลอดภัย
- SSL/TLS Encryption: การใช้ SSL/TLS เพื่อเข้ารหัสการสื่อสารระหว่าง Frontend และ Backend
- Data Validation: การตรวจสอบและกันการป้องกันข้อมูลที่ไม่ถูกต้องและการโจมตี SQL Injection
การใช้ ASP.NET Core ในบทบาทของ Backend สำหรับ Frontend ที่สร้างด้วย JavaScript Frameworks มีประสิทธิภาพสูงและช่วยในการพัฒนาแอปพลิเคชันที่มีประสิทธิภาพ ง่ายต่อการบำรุงรักษา และปลอดภัย การสร้างแอปพลิเคชันที่มี Backend และ Frontend ที่ทำงานร่วมกันโดยมีการสื่อสารผ่าน API เป็นแนวทางที่สมบูรณ์แบบสำหรับการพัฒนาซอฟต์แวร์ในยุคปัจจุบัน
11. การจัดการ Configuration
การจัดการ Configuration ใน ASP.NET Core เป็นกระบวนที่สำคัญที่ช่วยให้เราสามารถกำหนดค่าที่เปลี่ยนแปลงได้ต่าง ๆ ของแอปพลิเคชันได้โดยไม่ต้องเปลี่ยนแก้โค้ดและรีคอมไพล์ นี่คือวิธีที่ ASP.NET Core ใช้ในการจัดการ Configuration
11.1 AppSettings.json: ในไฟล์ appsettings.json สามารถกำหนดค่าต่าง ๆ ที่แอปพลิเคชันต้องการ
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"AppSettings": {
"ApiKey": "your-api-key",
"MaxItemCount": 100
}
}
...
11.2 Environment-specific Configuration: สามารถสร้างไฟล์ appsettings สำหรับ environment ที่ต่าง ๆ เช่น appsettings.Development.json, appsettings.Production.json เพื่อกำหนดค่าสำหรับแต่ละ environment
// appsettings.Development.json
{
"AppSettings": {
"ApiKey": "dev-api-key"
}
}
...
11.3 User Secrets: สามารถใช้ User Secrets เพื่อเก็บค่าที่ไม่ควรแชร์ในระหว่างทีมพัฒนา
dotnet user-secrets set "AppSettings:ApiKey" "your-secret-api-key"
...
11.4 Command Line Configuration: สามารถใช้ command line arguments เพื่อ override ค่า configuration ในระหว่างการรันแอปพลิเคชัน
dotnet run --AppSettings:MaxItemCount=50
...
11.5 Environment Variables: สามารถใช้ environment variables เพื่อกำหนดค่า configuration
export AppSettings ApiKey = "your-api-key"
...
11.6 Strongly Typed Configuration: ใน ASP.NET Core สามารถใช้ strongly typed configuration โดยสร้าง class ที่สร้าง mapping กับ configuration section ใน appsettings.json
public class AppSettings
{
public string ApiKey { get; set; }
public int MaxItemCount { get; set; }
}
public void ConfigureServices(IServiceCollection services)
{
services.Configure<AppSettings>(Configuration.GetSection("AppSettings"));
}
...
11.7 Configuration Providers: ASP.NET Core มี configuration providers ที่สามารถให้บริการได้หลายแหล่ง เช่น JSON, environment variables, command line arguments, Azure Key Vault, AWS Systems Manager Parameter Store และอื่น ๆ
public void ConfigureAppConfiguration(WebHostBuilderContext hostingContext, IConfigurationBuilder config)
{
config.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddJsonFile($"appsettings.{hostingContext.HostingEnvironment.EnvironmentName}.json", optional: true, reloadOnChange: true)
.AddEnvironmentVariables();
}
...
11.8 Configuration Reload: ASP.NET Core รองรับการ reload ของ configuration โดย default โดยการให้บริการ IOptionsMonitor<T>
public class MyService
{
private readonly AppSettings _appSettings;
public MyService(IOptionsMonitor<AppSettings> options)
{
_appSettings = options.CurrentValue;
options.OnChange(updatedSettings =>
{
// Handle configuration change
_appSettings = updatedSettings;
});
}
}
...
การจัดการ Configuration ใน ASP.NET Core ช่วยให้เราสามารถทำการตั้งค่าและกำหนดค่าของแอปพลิเคชันได้ในทุกระดับ โดยให้ความยืดหยุ่นและความสะดวกสบายในการบำรุงรักษาและการให้บริการ
ยังไม่ได้อ่าน EP1 กดตรงนี้ The basics of ASP.NET Core [EP1/2]