HTTP Status Codes
HTTP Status Codes เป็นรหัสสถานะที่เซิร์ฟเวอร์ส่งกลับมายังไคลเอนต์ (เช่น เว็บเบราว์เซอร์หรือโปรแกรมอื่น ๆ) เพื่อแสดงผลสถานะของการร้องขอ HTTP (HTTP Request)
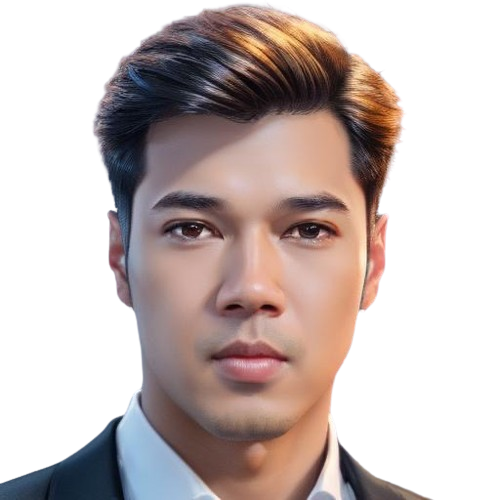
HTTP Status Codes
HTTP Status Codes เป็นรหัสสถานะที่เซิร์ฟเวอร์ส่งกลับมายังไคลเอนต์ (เช่น เว็บเบราว์เซอร์หรือโปรแกรมอื่น ๆ) เพื่อแสดงผลสถานะของการร้องขอ HTTP (HTTP Request) แต่ละสถานะมีความหมายเฉพาะเจาะจง และถูกแบ่งออกเป็น 5 กลุ่มหลัก ๆ ดังนี้
-
1. Informational Responses (รหัสสถานะ 1xx) รหัสสถานะกลุ่มนี้แสดงว่าคำร้องขอได้รับแล้ว และกำลังดำเนินการอยู่
- 100 Continue: ไคลเอนต์ควรดำเนินการส่งคำร้องขอต่อไป (ใช้ในขั้นตอนแรกของคำร้องขอแบบ multipart)
- 101 Switching Protocols: เซิร์ฟเวอร์กำลังเปลี่ยนโปรโตคอลตามคำขอของไคลเอนต์
-
-
2. Successful Responses (รหัสสถานะ 2xx) รหัสสถานะกลุ่มนี้แสดงว่าคำร้องขอประสบความสำเร็จและเซิร์ฟเวอร์ได้ส่งคำตอบที่ร้องขอกลับไป
- 200 OK: คำร้องขอสำเร็จและเซิร์ฟเวอร์ได้ส่งข้อมูลที่ร้องขอกลับไป
- 201 Created: คำร้องขอสำเร็จและมีการสร้างทรัพยากรใหม่
- 204 No Content: คำร้องขอสำเร็จแต่ไม่มีเนื้อหาที่จะส่งกลับมา
-
-
3. Redirection Messages (รหัสสถานะ 3xx) รหัสสถานะกลุ่มนี้แสดงว่าต้องการการกระทำเพิ่มเติมจากไคลเอนต์เพื่อให้คำร้องขอสำเร็จ
- 301 Moved Permanently: ทรัพยากรถูกย้ายไปยัง URL ใหม่อย่างถาวร
- 302 Found: ทรัพยากรถูกย้ายไปยัง URL ใหม่ชั่วคราว
- 304 Not Modified: ทรัพยากรไม่ได้ถูกแก้ไขตั้งแต่การร้องขอครั้งก่อน (ใช้สำหรับ caching)
-
-
4. Client Error Responses (รหัสสถานะ 4xx) รหัสสถานะกลุ่มนี้แสดงว่ามีข้อผิดพลาดจากฝั่งไคลเอนต์
- 400 Bad Request: คำร้องขอไม่ถูกต้องหรือไม่สามารถประมวลผลได้
- 401 Unauthorized: การร้องขอต้องการการยืนยันตัวตน
- 403 Forbidden: เซิร์ฟเวอร์ปฏิเสธการร้องขอถึงแม้ว่าการยืนยันตัวตนถูกต้อง
- 404 Not Found: ไม่พบทรัพยากรที่ร้องขอ
-
-
5. Server Error Responses (รหัสสถานะ 5xx) รหัสสถานะกลุ่มนี้แสดงว่ามีข้อผิดพลาดจากฝั่งเซิร์ฟเวอร์
- 500 Internal Server Error: เซิร์ฟเวอร์เกิดข้อผิดพลาดภายในที่ไม่ทราบสาเหตุ
- 501 Not Implemented: เซิร์ฟเวอร์ไม่รองรับฟังก์ชันที่ร้องขอ
- 503 Service Unavailable: เซิร์ฟเวอร์ไม่สามารถให้บริการได้ชั่วคราว
ตัวอย่างการใช้งาน HTTP Status Codes ใน C# Minimal API
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
var builder = WebApplication.CreateBuilder(args);
var app = builder.Build();
// In-memory storage for the sake of example
var items = new List-
{
new Item { Id = 1, Name = "Item1" },
new Item { Id = 2, Name = "Item2" }
};
// Get all items
app.MapGet("/api/items", () =>
{
return Results.Ok(items); // 200 OK
});
// Get an item by ID
app.MapGet("/api/items/{id}", (int id) =>
{
var item = items.FirstOrDefault(i => i.Id == id);
if (item == null)
{
return Results.NotFound(); // 404 Not Found
}
return Results.Ok(item); // 200 OK
});
// Create a new item
app.MapPost("/api/items", (Item newItem) =>
{
newItem.Id = items.Count > 0 ? items.Max(i => i.Id) + 1 : 1;
items.Add(newItem);
return Results.Created($"/api/items/{newItem.Id}", newItem); // 201 Created
});
// Update an existing item
app.MapPut("/api/items/{id}", (int id, Item updatedItem) =>
{
var item = items.FirstOrDefault(i => i.Id == id);
if (item == null)
{
return Results.NotFound(); // 404 Not Found
}
item.Name = updatedItem.Name;
return Results.NoContent(); // 204 No Content
});
// Delete an item
app.MapDelete("/api/items/{id}", (int id) =>
{
var item = items.FirstOrDefault(i => i.Id == id);
if (item == null)
{
return Results.NotFound(); // 404 Not Found
}
items.Remove(item);
return Results.NoContent(); // 204 No Content
});
app.Run();
record Item
{
public int Id { get; set; }
public string Name { get; set; }
}
-
อธิบายการทำงานของโค้ด
- GET /api/items: ดึงรายการทั้งหมดของ items. ส่งกลับสถานะ 200 OK พร้อมข้อมูลรายการ
- GET /api/items/{id}: ดึงข้อมูล item ตาม ID ที่ระบุ. ถ้าไม่พบ item ส่งกลับสถานะ 404 Not Found, ถ้าพบ ส่งกลับสถานะ 200 OK พร้อมข้อมูล item
- POST /api/items: สร้าง item ใหม่. กำหนด ID ให้ item ใหม่และเพิ่มลงในรายการ items. ส่งกลับสถานะ 201 Created พร้อมข้อมูล item ที่สร้างใหม่
- PUT /api/items/{id}: อัปเดต item ที่มีอยู่ตาม ID ที่ระบุ. ถ้าไม่พบ item ส่งกลับสถานะ 404 Not Found, ถ้าพบ ส่งกลับสถานะ 204 No Content หลังจากการอัปเดต
- DELETE /api/items/{id}: ลบ item ตาม ID ที่ระบุ. ถ้าไม่พบ item ส่งกลับสถานะ 404 Not Found, ถ้าพบ ส่งกลับสถานะ 204 No Content หลังจากการลบ
นี่คือตัวอย่างการสร้าง RESTful API ด้วย Minimal APIs ใน .NET 6 ขึ้นไป ซึ่งแสดงให้เห็นการใช้ HTTP methods และ status codes อย่างถูกต้องตามหลักการของ RESTful API Minimal APIs ช่วยให้การเขียน API มีความเรียบง่ายและกระชับมากขึ้น ทำให้สามารถพัฒนา API ได้อย่างรวดเร็วและมีประสิทธิภาพ
ตัวอย่างการใช้งาน HTTP Status Codes ใน C# Web API ด้วย ASP.NET Core
namespace suwaphan.Models
{
public class Item
{
public int Id { get; set; }
public string Name { get; set; }
}
}
using Microsoft.AspNetCore.Mvc;
using suwaphan.Models;
using System.Collections.Generic;
using System.Linq;
namespace suwaphan.Controllers
{
[ApiController]
[Route("api/[controller]")]
public class ItemsController : ControllerBase
{
// In-memory storage for the sake of example
private static List<Item> items = new List<Item>
{
new Item { Id = 1, Name = "Item1" },
new Item { Id = 2, Name = "Item2" }
};
// GET: api/items
[HttpGet]
public ActionResult<IEnumerable<Item>> GetItems()
{
return Ok(items); // 200 OK
}
// GET: api/items/1
[HttpGet("{id}")]
public ActionResult<Item> GetItem(int id)
{
var item = items.FirstOrDefault(i => i.Id == id);
if (item == null)
{
return NotFound(); // 404 Not Found
}
return Ok(item); // 200 OK
}
// POST: api/items
[HttpPost]
public ActionResult<Item> PostItem(Item newItem)
{
newItem.Id = items.Count > 0 ? items.Max(i => i.Id) + 1 : 1;
items.Add(newItem);
return CreatedAtAction(nameof(GetItem), new { id = newItem.Id }, newItem); // 201 Created
}
// PUT: api/items/1
[HttpPut("{id}")]
public IActionResult PutItem(int id, Item updatedItem)
{
var item = items.FirstOrDefault(i => i.Id == id);
if (item == null)
{
return NotFound(); // 404 Not Found
}
item.Name = updatedItem.Name;
return NoContent(); // 204 No Content
}
// DELETE: api/items/1
[HttpDelete("{id}")]
public IActionResult DeleteItem(int id)
{
var item = items.FirstOrDefault(i => i.Id == id);
if (item == null)
{
return NotFound(); // 404 Not Found
}
items.Remove(item);
return NoContent(); // 204 No Content
}
}
}
-
อธิบายการทำงานของโค้ด
- GET /api/items: ดึงรายการทั้งหมดของ items. ส่งกลับสถานะ 200 OK พร้อมข้อมูลรายการ
- GET /api/items/{id}: ดึงข้อมูล item ตาม ID ที่ระบุ. ถ้าไม่พบ item ส่งกลับสถานะ 404 Not Found, ถ้าพบ ส่งกลับสถานะ 200 OK พร้อมข้อมูล item
- POST /api/items: สร้าง item ใหม่. กำหนด ID ให้ item ใหม่และเพิ่มลงในรายการ items. ส่งกลับสถานะ 201 Created พร้อมข้อมูล item ที่สร้างใหม่
- PUT /api/items/{id}: อัปเดต item ที่มีอยู่ตาม ID ที่ระบุ. ถ้าไม่พบ item ส่งกลับสถานะ 404 Not Found, ถ้าพบ ส่งกลับสถานะ 204 No Content หลังจากการอัปเดต
- DELETE /api/items/{id}: ลบ item ตาม ID ที่ระบุ. ถ้าไม่พบ item ส่งกลับสถานะ 404 Not Found, ถ้าพบ ส่งกลับสถานะ 204 No Content หลังจากการลบ
ตัวอย่างนี้แสดงการสร้าง RESTful API โดยใช้ Controller-based approach ใน ASP.NET Core ซึ่งจะให้โครงสร้างที่ชัดเจนและเป็นไปตามมาตรฐานของ RESTful API เราสามารถขยายและปรับปรุงเพิ่มเติมตามความต้องการของโปรเจคของเราได้ง่าย ๆ ด้วยการเพิ่ม Model, Controller, และ Method ตามหลักการของ MVC
การใช้งาน HTTP Status Codes อย่างถูกต้องจะช่วยให้ API ของเรามีความชัดเจนและเป็นไปตามมาตรฐาน ทำให้ผู้ใช้สามารถเข้าใจและจัดการกับการตอบสนองจากเซิร์ฟเวอร์ได้อย่างถูกต้อง